Python进阶之pillow模块绘制验证码
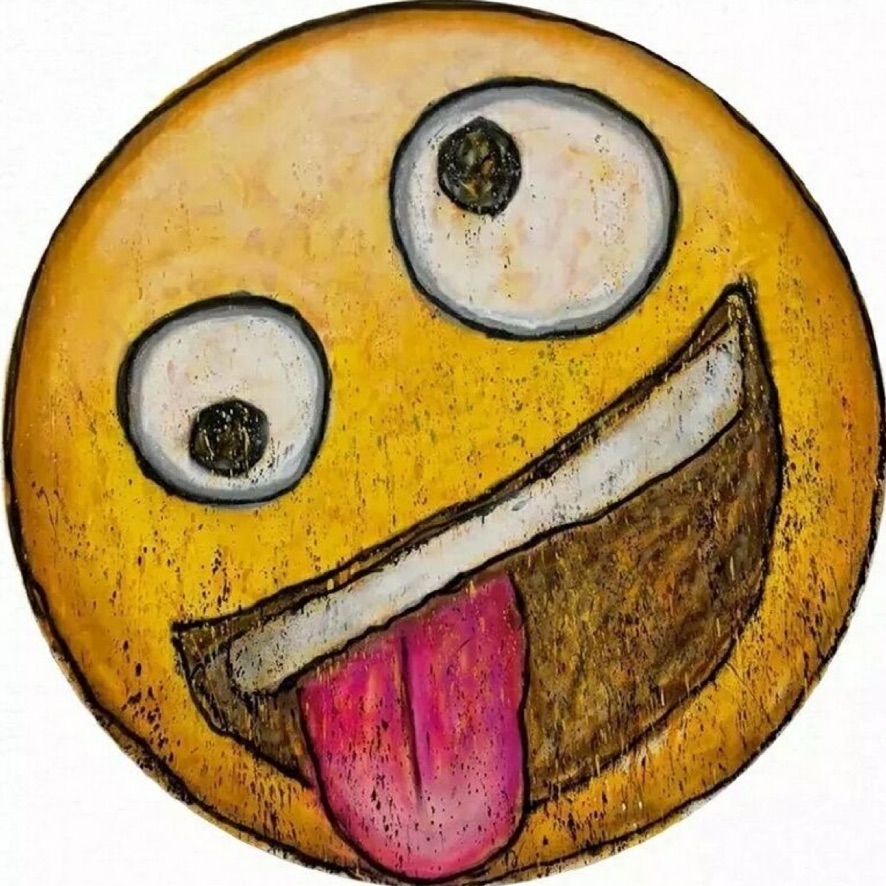
简介
PIL:Python Imaging Library,是一个强大的图像处理标准库,Pillow 是由 Python2.x 版本中PIL的基础上延伸来的更加兼容的版本。
Pillow 模块主要提供了图像处理的功能,可以处理图像、做图像缩放、旋转、翻转、裁剪、调整亮度与对比度等操作,支持多种图像格式,支持图像的滤镜处理,支持高级图像处理,支持图片编码和解码,支持图像和文本的混合输出,支持多平台,可派生到多种语言。
安装和导入
安装Pillow模块:
pip install pillow
:
导入Pillow:
from PIL import xxx
快速上手
1 | from PIL import Image, ImageDraw, ImageFont, ImageFilter |
** **
实战
日常Web 开发过程中,在注册及登录验证时,数字验证码使用场景颇多。虽然得益于 Django 强大的第三方库如:
- django-simple-captcha:支持生成简单的文字验证码和数学验证码,支持中文。
- django-captcha:支持生成简单的文字验证码,支持自定义字体大小、字体类型等。
- django-recaptcha:基于Google reCAPTCHA,支持验证码和滑动验证码,支持多种语言。
- django-simple-captcha-update:支持生成简单的文字验证码,支持中文,支持自定义字体大小等。
但通过 Pillow 模块我们自己也能实现简单的数字验证码功能。
演示如下:
1 | import random |
效果预览
根据个人需求还可以增加随机字体大小,模糊背景等功能,更多使用方法参考Pillow 官方文档
评论